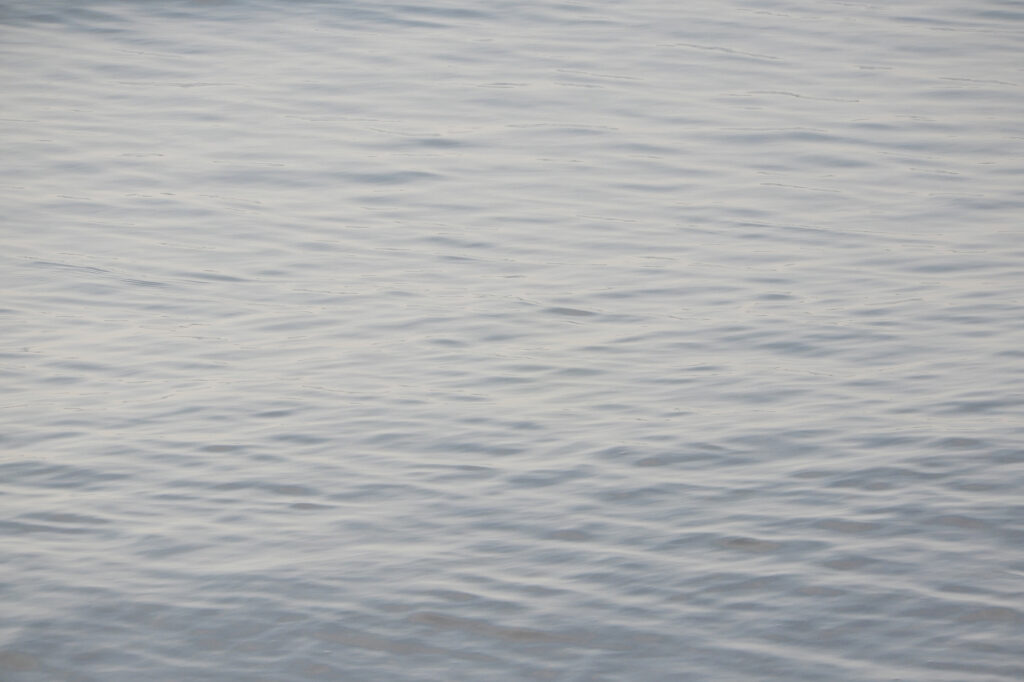
BLOG the POST
- CATEGORYXX
-
CATEGORY↓ scroll
- ARCHIVEX
-
ARCHIVE↓ scroll
- TOPICX
Title: click や touch で画像の拡大 | jQuery v3
|
topic:jQuery ver 3 での click や touch 操作による「シンプルな画像拡大」のコード紹介です。
今回は、あまり一般的ではありませんが、<img> に「sizes=””」を付与し最大最小サイズが制約されている際に適応し難い拡大処理を実行する方法です。モニターサイズに合わせて拡大や縮小をし画像全体を表示させます。
上述の制約がない場合は、数行のCSSとjQueryで実行可能な内容ですので悪しからず…。
実装サンプル.
要旨.
□ 記述ファイル:HTML・ CSS・JS
□ 画像にaタグは付けない( figureタグで囲う前提。置換コード後述。 )
□ 拡大画像ハミだし回避( 自動調整 )
□ 画像の縦幅や横幅を基準に拡大率を算出
HTMLコード.
上記HTMLコードは、ラップ容量の大きなブロック要素の外側に配置すると表示崩れなどのトラブルがありません。
2行目「 class=”large_img” 」の中に拡大用画像が複製されます。
3行目「 class=”overlay_modal” 」 は拡大時の背景です。
拡大画像もしくは背景を クリック or タッチ すれば、拡大を閉じる仕様です。必要な場合は、Close ボタンを設置されると良いかと思います。
CSSコード.
.modal {
display: none;
width: 100%;
height: 100vh;
position: fixed;
top: 0;
left: 0;
right: 0;
bottom: 0;
z-index: 1999;
}
.overlay_modal {
width: 100%;
height: 100vh;
margin: auto;
position: fixed;
top: 0;
left: 0;
right: 0;
bottom: 0;
z-index: 1999;
background: rgba(0, 0, 0, 0.8);
}
.large_img {
cursor: pointer;
/* for center layout */
display: grid;
place-items: center;
/* for center layout end */
max-width: 80%; /* 拡大画像の中央表示と余白バランス調整用 */
height: auto;
margin: auto;
position: fixed;
top: 0;
left: 0;
right: 0;
bottom: 0;
z-index: 2000;
}
.large_img img {
position: absolute;
margin: auto;
top: 0;
left: 0;
right: 0;
bottom: 0;
}
上記30行目は、中央配置と余白バランス用の記述ですが拡大画像サイズにも影響します。
hover 時の画像の透過は以下のような感じに。
article.press img {
cursor: pointer;
opacity: 1;
transition: 0.6s ease-in-out;
}
article.press img:hover {
opacity: 0.6;
}
JSコード.
画像の縦横サイズ判定を行い、モニターサイズに合わせて拡大値を算出する内容です。
横長、縦長、正方形、の画像を想定しています。
// img 拡大表示用( 自動伸縮 ) ※ touchend NG
jQuery('article.press figure').each(function(){
jQuery('.modal').hide();
jQuery('.large_img').hide();
jQuery(this).on('click',function(){
var img_def = jQuery(this).children().clone();
jQuery('.large_img').append(img_def);
//モニター用サイズ判定 拡大規制
var wh = window.innerWidth;
var ht = window.innerHeight;
var chn_wh = jQuery(this).children().width() + 10; // + 10px adjust
var chn_ht = jQuery(this).children().height() + 10; // + px ※ 同値でないと算出影響
var scl_w = parseFloat((wh / chn_wh).toFixed(2)); // 小数点2桁に指定
var scl_h = parseFloat((ht / chn_ht).toFixed(2));
var scl = 1.5; // 最大拡大比率
if(chn_wh > chn_ht){
jQuery('.large_img img').addClass('w_type'); // class → 元画像へのscale値の転移回避
if(wh < 835 && wh > ht){
// smart phone & tablet 横向き時のみ更に指定 ※ 横幅基準から縦幅基準へ変更
if(scl_h < scl){
jQuery('img.w_type').css({transform: 'scale('+ scl_h +')'});
} else {
jQuery('img.w_type').css({transform: 'scale('+ scl +')'});
}
} else {
if(scl_w < scl){
jQuery('img.w_type').css({transform: 'scale('+ scl_w +')'});
} else {
jQuery('img.w_type').css({transform: 'scale('+ scl +')'});
}
}
}
if(chn_wh < chn_ht){
jQuery('.large_img img').addClass('h_type');
if(scl_h < scl){
jQuery('img.h_type').css({transform: 'scale('+ scl_h +')'});
} else {
jQuery('img.h_type').css({transform: 'scale('+ scl +')'});
}
}
if(chn_wh == chn_ht){
jQuery('.large_img img').addClass('s_type');
if(wh < 835 && wh > ht){
// smart phone & tablet 横向き時のみ更に指定 ※ 横幅基準から縦幅基準へ変更
if(scl_h < scl){
jQuery('img.s_type').css({transform: 'scale('+ scl_h +')'});
} else {
jQuery('img.s_type').css({transform: 'scale('+ scl +')'});
}
} else {
if(scl_w < scl){
jQuery('img.s_type').css({transform: 'scale('+ scl_w +')'});
} else {
jQuery('img.s_type').css({transform: 'scale('+ scl +')'});
}
}
}
//モニター用サイズ判定 拡大規制 end
jQuery('.modal').fadeIn();
jQuery('.large_img').fadeIn(1000);
jQuery('html').css({'overflow':'hidden'}); // scroll禁止
return true;
});
//overlay ( close ) , .close-btn
jQuery('.overlay_modal , .large_img').on('click touchend',function(){
jQuery('.large_img').fadeOut(300).empty();
jQuery('.modal').fadeOut(500);
jQuery('html').css({'overflow':'visible'}); // scroll禁止の解除
return true;
});
});
11行目、12行目の「 + 10 」は、拡大画像がモニター幅ぴったりになるのを防ぐ目的で、加算しています。
18行目、43行目は、スマホやタブレットの横向き時のために拡大値を再算出しています
横長モニター時: モニター縦幅 ÷ 画像縦幅
縦長モニター時: モニター横幅 ÷ 画像横幅
という基準で拡大値を算出しています。
補足事項.
今回は、imgの親要素を<figure>と想定していますが、imgの親要素<a>を削除する場合や<figure>に置換する場合は、下記コードのような感じでリプレイスされると良いかと思います。
// imgの親要素がaの場合削除
let img_a = jQuery('article.press img').parents('a');
jQuery(img_a).replaceWith(function() {
jQuery(this).replaceWith(""+jQuery(this).html()+"");
});
今回は、画像サイズ取得と拡大比率の算出が必要なため jQuery 側で、img に直接 CSS を指定させていますが、実務的には、CSS 側で処理 ( addClassでクラス付与や、removeClassで解除 ) させた方が、読込みが速いので、可能な限り、CSS側での記述をお奨めします。
- POINT
-
- JS側よりCSS側で記述する方が処理速し。SEO施策的にも良し。
- jQuery v3の場合「 jQuery(window).on( ‘load’, function (){} ); 」内で実行してあげると、画像の縦横判定や初回読込みが正常に実行されます。初回読込み時に判定が必要な場合は「 jQuery( function ( ){ } ); 」内で実行しないこと。( 画像サイズ取得など不安定に )
- 「 jQuery(window).on( ‘load’, function (){} ); 」は1ファイル1箇所を基本に。多用すると正常に動作しません。
最大拡大比率 1.5 の設定理由.
重ねてになりますが、本記事の内容は、<img> に「sizes=””」を付与し最大最小サイズが制約されている際に適応し難い拡大処理を実行する方法です。
その上で、表題の最大拡大比率に設定した理由は、一般的なモニターサイズのノートパソコンでWEBサイトを閲覧すると、モニター縦幅は、実質640px程度。写真の縦横比率は基本的に3:2。3÷2=1.5。今回は、そのことを考慮して、デスクトップやノートPCに配置される縦長画像は、最大 height: 640px と想定。横幅426.66..px。
640px を1.5倍すると960px。426.66pxを1.5倍すると639.99px。拡大値の基準としては、モニターサイズと画像品質を鑑みて、この程度が最適だとしました。
モニター比率を16:9と想定して拡大値1.77としても良いかと思いますし、サイト設計( 設置画像の平均サイズ )に合わせて、お好みで基準となる数値を設定されると良いかと思います。
拡大画像が、モニターからハミでる場合は、モニターサイズに合わせて縮小表示されます。
注)拡大値を大きく設定しすぎると、スマホやタブレットのモニター幅からハミでる場合がありますので適宜ご調整を。
さいごに.
ご読了いただき有難う御座います。
不束なコードがあればご容赦下さい。
<img> に「sizes=””」を付与し最大最小サイズが制約されている際に「 jQuery ver3 での click や touch 操作による画像の拡大 」でした。
お役に立てば幸いです。
author: Kouzou Saika
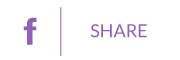
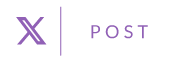
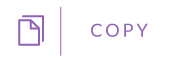